Can I use Python libraries in Domo?
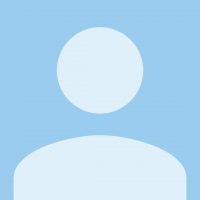
DataLawton
Member
in Magic ETL
Comments
-
You can view a list of installed packages on the packages tab inside the python tile. If you do a search for scipy you should see it in the list.
1 -
Make sure scipy.optimize is installed. It should be pre-installed in the Domo Python environment.
import pandas as pd
from scipy.optimize import minimizeThe Python tile will use Pandas DataFrames. Something like this.
import pandas as pd
from scipy.optimize import minimize
# Assuming your input data looks something like this:
# df = pd.DataFrame({
# 'variable': ['x1', 'x2'],
# 'coefficient': [5, 8],
# 'constraint': [20, 30]
# })
# Input data from the Python Tile
coefficients = df['coefficient'].values
constraints = df['constraint'].values
# Objective function to minimize
def objective(x):
return sum(coefficients * x)
# Constraints: Example constraint that the sum of variables <= 100
constraint = {'type': 'ineq', 'fun': lambda x: 100 - sum(x)}
# Bounds: Non-negative variables
bounds = [(0, None)] * len(coefficients)
# Initial guess for the variables
x0 = [0] * len(coefficients)
# Run the optimization
result = minimize(objective, x0, bounds=bounds, constraints=[constraint])
# Output results
df_output = pd.DataFrame({'variable': df['variable'], 'value': result.x})Note - Domo also has Jupyter Workspace, where you can use Python code.
** Was this post helpful? Click Agree or Like below. **
** Did this solve your problem? Accept it as a solution! **0
Categories
- All Categories
- 2K Product Ideas
- 2K Ideas Exchange
- 1.6K Connect
- 1.3K Connectors
- 311 Workbench
- 7 Cloud Amplifier
- 9 Federated
- 3K Transform
- 114 SQL DataFlows
- 654 Datasets
- 2.2K Magic ETL
- 4.1K Visualize
- 2.5K Charting
- 806 Beast Mode
- 80 App Studio
- 44 Variables
- 761 Automate
- 189 Apps
- 480 APIs & Domo Developer
- 75 Workflows
- 17 DomoAI
- 40 Predict
- 17 Jupyter Workspaces
- 23 R & Python Tiles
- 408 Distribute
- 119 Domo Everywhere
- 279 Scheduled Reports
- 10 Software Integrations
- 141 Manage
- 137 Governance & Security
- 8 Domo Community Gallery
- 47 Product Releases
- 12 Domo University
- 5.4K Community Forums
- 41 Getting Started
- 31 Community Member Introductions
- 114 Community Announcements
- 4.8K Archive