Errors when using domolibrary with client ID and secret
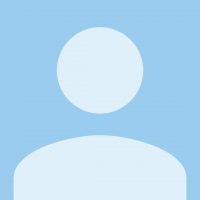
Background and setup: I created a client using developer.domo.com and gave it the "data" scope. I have a client ID and secret now. I have tested it using the PyDomo library and it has worked. However, my goal is to programmatically get and store dataflows as an off-Domo backup. Domolibrary was recommended in another thread. I'm trying to use DomoDeveloperAuth in domolibrary, as it appears to allow use of client ID and secret. I'm following this snippet.
Problem: I'm not all that familiar with await— I'm trying to learn more Python at the same time— but it appears to require the asyncio library and applies to functions, like this:
import asyncio
async def my_function():
response = await <call that we need to wait for>
return response
So, perhaps incorrectly, I assumed that the code in the snippet needs to be placed in a function, like this:
import asyncio
import domolibrary.client.DomoAuth as dmda
async def fetch_auth_token(domo_client_id, domo_client_secret):
try:
domo_auth = dmda.DomoDeveloperAuth(
domo_client_id=domo_client_id,
domo_client_secret=domo_client_secret)
await domo_auth.get_auth_token()
except InvalidCredentialsError as e:
print(e)
domo_client_id = '<my_client_id>'
domo_client_secret = '<my_client_secret>'
asyncio.run(fetch_auth_token(domo_client_id, domo_client_secret))
When I run that, I get the error: "AttributeError: 'DomoDeveloperAuth' object has no attribute 'set_manual_login'. Did you mean: 'url_manual_login'?
". I don't know where to go from there. Is domolibrary using a deprecated API option? Do I have this set up completely wrong? Thanks in advance for any pointers.
Best Answer
-
Thanks guys.
Yeah, you have to use DomoTokenAuth to move forward. most of this library uses undocumented APIs which require the use of an Access_Token (made in Domo > Admin > Access Tokens) client_id and secret only works with public APIs.
if you look in the documentation, there's tons of working code for almost each method that has been implemented
"However, my goal is to programmatically get and store dataflows as an off-Domo backup"this is cake.you'll need a
auth = dmda.DomoTokenAuth(…) # could also use FullAuth which is username and password authentication res = await DomoDatacenter.search_datacenter( auth=token_auth, entity_type=datacenter_routes.Datacenter_Enum.DATAFLOW.value, )
then just follow this tutorial
Jae Wilson
Check out my 🎥 Domo Training YouTube Channel 👨💻
**Say "Thanks" by clicking the ❤️ in the post that helped you.
**Please mark the post that solves your problem by clicking on "Accept as Solution"1
Answers
-
Based on the error, it looks like "set_manual_login" isn't a valid object in DomoDeveloperAuth. Have you tried the "url_manual_login" like it suggests?
0 -
Thanks for the reply, and sorry for not responding sooner. I did try specifying url_manual_login explicitly, although I don't actually have a manual login to use. So, I did url_manual_login = None. That didn't help. The problem appears to be that I've not used set_manual_login and yet it is assumed. If you see my badly-formatted code above, you'll see that I don't use that option.
As I review the docs for domolibrary I wonder if I've stumbled onto a project under development. It's looking more and more like I should move on.
0 -
@jaeW_at_Onyx would be the one best suited to help you on this.
**Check out my Domo Tips & Tricks Videos
**Make sure toany users posts that helped you.
**Please mark as accepted the ones who solved your issue.0 -
Thanks guys.
Yeah, you have to use DomoTokenAuth to move forward. most of this library uses undocumented APIs which require the use of an Access_Token (made in Domo > Admin > Access Tokens) client_id and secret only works with public APIs.
if you look in the documentation, there's tons of working code for almost each method that has been implemented
"However, my goal is to programmatically get and store dataflows as an off-Domo backup"this is cake.you'll need a
auth = dmda.DomoTokenAuth(…) # could also use FullAuth which is username and password authentication res = await DomoDatacenter.search_datacenter( auth=token_auth, entity_type=datacenter_routes.Datacenter_Enum.DATAFLOW.value, )
then just follow this tutorial
Jae Wilson
Check out my 🎥 Domo Training YouTube Channel 👨💻
**Say "Thanks" by clicking the ❤️ in the post that helped you.
**Please mark the post that solves your problem by clicking on "Accept as Solution"1 -
Thank you for the reply @jaeW_at_Onyx. Just to make sure I have the right idea, here's what I think I need to do, in very general terms. Since my org is using SSO, I am forced to use client ID and secret.
- Log in to Domo using client ID and secret acquired from developer.domo.com. This means using DomoDeveloperAuth.
- Once logged in, acquire an access token using DomoTokenAuth.
- Use that token as auth to use DomoDatacenter.
Step 1 should be this, then:
import asyncio import domolibrary.client.DomoAuth as dmda async def fetch_auth_token(domo_client_id, domo_client_secret): try: domo_auth = dmda.DomoDeveloperAuth( domo_client_id=domo_client_id, domo_client_secret=domo_client_secret) await domo_auth.get_auth_token() except InvalidCredentialsError as e: print(e) domo_client_id = '<my_client_id>' ## Generated at developer.domo.com domo_client_secret = '<my_client_secret>' ## Generated at developer.domo.com asyncio.run(fetch_auth_token(domo_client_id, domo_client_secret))
That's a code snippet from the docs, but wrapped in
async
so thatawait
will work. As far as that goes, should it work?0 -
no. you cannot use DeveloperAuth AND hit undocumented APIs.
you must use TokenAuth.
Jae Wilson
Check out my 🎥 Domo Training YouTube Channel 👨💻
**Say "Thanks" by clicking the ❤️ in the post that helped you.
**Please mark the post that solves your problem by clicking on "Accept as Solution"1
Categories
- All Categories
- 1.8K Product Ideas
- 1.8K Ideas Exchange
- 1.5K Connect
- 1.2K Connectors
- 300 Workbench
- 6 Cloud Amplifier
- 8 Federated
- 2.9K Transform
- 100 SQL DataFlows
- 616 Datasets
- 2.2K Magic ETL
- 3.9K Visualize
- 2.5K Charting
- 738 Beast Mode
- 57 App Studio
- 40 Variables
- 685 Automate
- 176 Apps
- 452 APIs & Domo Developer
- 47 Workflows
- 10 DomoAI
- 36 Predict
- 15 Jupyter Workspaces
- 21 R & Python Tiles
- 394 Distribute
- 113 Domo Everywhere
- 275 Scheduled Reports
- 6 Software Integrations
- 124 Manage
- 121 Governance & Security
- 8 Domo Community Gallery
- 38 Product Releases
- 10 Domo University
- 5.4K Community Forums
- 40 Getting Started
- 30 Community Member Introductions
- 108 Community Announcements
- 4.8K Archive