Import CSV File via Python (Domo API)
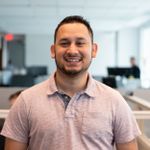
Hello, community,
I am currently writing a python app that will allow me to import CSV data (per the requirements of the developer.domo.com API reference).
When uploading the CSV file via the python SDK and/or using PUT command, I get a 400 error.
Error:
Error uploading DataSet: {"status":400,"statusReason":"Bad Request","message":"Only DataSets created with the API can be updated via APIs.","toe":"7RSBGISL15-SPKQ3-FBFG0"}
Question:
Has anyone ever been able to pass in the CSV file as it is (file path) either using the SDK or with the PUT request in the content-type or body?
Logically, being able to pass the file would make sense, but, what I am guessing (documentation is lacking here on Domo end) that they prefer not to handle unpacking a file via a request -> loading data -> discarding file.
If you have successfully been able to pass the data in without the file path could you kindly share with me an example successful post request?
The dataset I am trying to append data to with the CSV file is this connector type. Is it possible this is the wrong connector (image below)?
Thank you in advance and I appreciate your wisdom!
Humbly,
Isaiah Melendez
Best Answer
-
The awkward moment when you figure it out and have to post the answer to your own question.
What I figured out:
- If you use the Python SDK, leverage the datasets.create() method to create an API driven dataset.
- You will need to instantiate the DataSetRequest() object and pass in your
- Build out your schema for your using DSR
- Execute dataset creation
- Leverage the data_import_from_file() method to upload the csv data to the newly created API dataset
def create_dataset(self): domo_client = self.generate_domo_client() dsr = DataSetRequest() dsr.name = 'ATME_TEST_PYTHON' dsr.description = 'This is a test' dsr.schema = Schema([Column(ColumnType.LONG, 'shop_id'), Column(ColumnType.STRING, 'location_id'), Column(ColumnType.LONG, 'status_id'), Column(ColumnType.DATETIME, 'date_closed'), Column(ColumnType.STRING, 'firstname'), Column(ColumnType.STRING, 'lastname'), Column(ColumnType.LONG, 'remote_customer_id'), Column(ColumnType.LONG, 'year'), Column(ColumnType.STRING, 'make'), Column(ColumnType.STRING, 'model'), Column(ColumnType.LONG, 'remote_vehicle_id'), Column(ColumnType.STRING, 'vin'), Column(ColumnType.STRING, 'license_plate'), Column(ColumnType.LONG, 'remote_ticket_id'), Column(ColumnType.LONG, 'ro_number'), Column(ColumnType.DATETIME, 'dvi_signoff_datetime'), Column(ColumnType.LONG, 'dvi_images'), Column(ColumnType.LONG, 'total_rvh_images'), Column(ColumnType.LONG, 'dvi_motovisuals'), Column(ColumnType.LONG, 'dvi_videos'), Column(ColumnType.LONG, 'dvi_notes'), Column(ColumnType.LONG, 'dvi_green_items'), Column(ColumnType.LONG, 'dvi_orange_items'), Column(ColumnType.LONG, 'dvi_red_items'), Column(ColumnType.LONG, 'dvi_recommendations'), Column(ColumnType.DATETIME, 'texted_on'), Column(ColumnType.DATETIME, 'emailed_on'), Column(ColumnType.DATETIME, 'viewed_on')]) dataset = domo_client.datasets.create(dataset_request=dsr) domo_client.logger.info("Created DataSet " + dataset['id']) return "creating dataset complete" def update_dataset(self): file_path = 'path\to\csvfile.csv' domo_client = self.generate_domo_client() domo_client.datasets.data_import_from_file(dataset_id=os.getenv("DOMO_TEST_DSID"), filepath=file_path, update_method='APPEND') return "updated dataset"
Hope this code solution works for you and helps you out.
0
Answers
-
The awkward moment when you figure it out and have to post the answer to your own question.
What I figured out:
- If you use the Python SDK, leverage the datasets.create() method to create an API driven dataset.
- You will need to instantiate the DataSetRequest() object and pass in your
- Build out your schema for your using DSR
- Execute dataset creation
- Leverage the data_import_from_file() method to upload the csv data to the newly created API dataset
def create_dataset(self): domo_client = self.generate_domo_client() dsr = DataSetRequest() dsr.name = 'ATME_TEST_PYTHON' dsr.description = 'This is a test' dsr.schema = Schema([Column(ColumnType.LONG, 'shop_id'), Column(ColumnType.STRING, 'location_id'), Column(ColumnType.LONG, 'status_id'), Column(ColumnType.DATETIME, 'date_closed'), Column(ColumnType.STRING, 'firstname'), Column(ColumnType.STRING, 'lastname'), Column(ColumnType.LONG, 'remote_customer_id'), Column(ColumnType.LONG, 'year'), Column(ColumnType.STRING, 'make'), Column(ColumnType.STRING, 'model'), Column(ColumnType.LONG, 'remote_vehicle_id'), Column(ColumnType.STRING, 'vin'), Column(ColumnType.STRING, 'license_plate'), Column(ColumnType.LONG, 'remote_ticket_id'), Column(ColumnType.LONG, 'ro_number'), Column(ColumnType.DATETIME, 'dvi_signoff_datetime'), Column(ColumnType.LONG, 'dvi_images'), Column(ColumnType.LONG, 'total_rvh_images'), Column(ColumnType.LONG, 'dvi_motovisuals'), Column(ColumnType.LONG, 'dvi_videos'), Column(ColumnType.LONG, 'dvi_notes'), Column(ColumnType.LONG, 'dvi_green_items'), Column(ColumnType.LONG, 'dvi_orange_items'), Column(ColumnType.LONG, 'dvi_red_items'), Column(ColumnType.LONG, 'dvi_recommendations'), Column(ColumnType.DATETIME, 'texted_on'), Column(ColumnType.DATETIME, 'emailed_on'), Column(ColumnType.DATETIME, 'viewed_on')]) dataset = domo_client.datasets.create(dataset_request=dsr) domo_client.logger.info("Created DataSet " + dataset['id']) return "creating dataset complete" def update_dataset(self): file_path = 'path\to\csvfile.csv' domo_client = self.generate_domo_client() domo_client.datasets.data_import_from_file(dataset_id=os.getenv("DOMO_TEST_DSID"), filepath=file_path, update_method='APPEND') return "updated dataset"
Hope this code solution works for you and helps you out.
0
Categories
- All Categories
- 1.8K Product Ideas
- 1.8K Ideas Exchange
- 1.5K Connect
- 1.2K Connectors
- 296 Workbench
- 6 Cloud Amplifier
- 8 Federated
- 2.9K Transform
- 100 SQL DataFlows
- 614 Datasets
- 2.2K Magic ETL
- 3.8K Visualize
- 2.5K Charting
- 729 Beast Mode
- 53 App Studio
- 40 Variables
- 677 Automate
- 173 Apps
- 451 APIs & Domo Developer
- 45 Workflows
- 8 DomoAI
- 34 Predict
- 14 Jupyter Workspaces
- 20 R & Python Tiles
- 394 Distribute
- 113 Domo Everywhere
- 275 Scheduled Reports
- 6 Software Integrations
- 121 Manage
- 118 Governance & Security
- Domo Community Gallery
- 32 Product Releases
- 10 Domo University
- 5.4K Community Forums
- 40 Getting Started
- 30 Community Member Introductions
- 108 Community Announcements
- 4.8K Archive