Variables with Python Forecasting
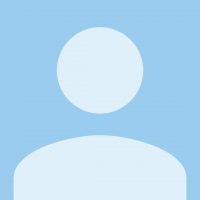
Is there a way to use variables with a Python forecast model like ARIMA or Random Forest?
I have a linear regression working, and I'm able to extract the coefficients, create variables, and use a Beast Mode calculation to generate a forecast. However, I'm unsure if this can be done with models that aren’t linear.
Ideally, I'd like the user to input their exogenous/explanatory variables and see a forecast at the dashboard level. Has anyone done something similar?
Best Answer
-
1. Model Setup with ARIMA (Exogenous Variables) or Random Forest
- ARIMA with Exogenous Variables (ARIMAX): ARIMA can accept external (exogenous) variables in addition to time series data. You can set up an ARIMAX model using Python in Domo's data science tool (like Jupyter Workspaces or Python tile).
- In Python, when fitting an ARIMAX model, use the
statsmodels
library'sSARIMAX
method, and pass your exogenous variables. - After training the model, you can store the forecast results in a dataset in Domo.
- In Python, when fitting an ARIMAX model, use the
- Random Forest for Forecasting: You can also use Random Forest Regressors (or other models like XGBoost) for forecasting if you have explanatory variables. After training the model in a Python tile, you can output the predictions to a dataset.
2. User Input for Exogenous Variables
- Webform for Input: You can create a Domo Webform for user input. This webform could capture variables like economic indicators, marketing spend, or any other factors that affect your forecast.
- These inputs will be stored in a dataset that can be dynamically updated as users enter new values.
- Domo Variables: Domo introduced a Variables feature that allows users to create variables on a dashboard and use them to dynamically adjust visualizations. You can create variables that correspond to the exogenous/explanatory variables used in your ARIMAX or Random Forest model. Users can interact with these variables using dropdowns, sliders, or text inputs on the dashboard.
3. Real-Time Forecast with Python Integration
- After obtaining user input (either through a webform or Domo Variables), you need to link these inputs back into your forecast model.
- In Python, you can fetch this data dynamically (e.g., pulling the most recent inputs from the webform dataset or variable inputs).
- Update your model or create a new prediction based on these inputs, then write the forecast results to a new dataset.
- This dataset can then be visualized on the dashboard, showing the forecast based on the user's custom inputs in real-time.
** Was this post helpful? Click Agree or Like below. **
** Did this solve your problem? Accept it as a solution! **0 - ARIMA with Exogenous Variables (ARIMAX): ARIMA can accept external (exogenous) variables in addition to time series data. You can set up an ARIMAX model using Python in Domo's data science tool (like Jupyter Workspaces or Python tile).
Answers
-
You should be able to use the Python tile to use Domo inputs or filtering. Run something like
from statsmodels.tsa.arima.model import ARIMA
y = your_time_series
X = your_exogenous_variable
model = ARIMA(y, exog=X, order=(p, d, q))
model_fit = model.fit()
forecast = model_fit.forecast(steps=10, exog=user_input_exog)** Was this post helpful? Click Agree or Like below. **
** Did this solve your problem? Accept it as a solution! **0 -
Um…I did not put those numbers on that code. Hmm.
** Was this post helpful? Click Agree or Like below. **
** Did this solve your problem? Accept it as a solution! **0 -
I have a dataset and the model working right now, but the problem is getting input on the dashboard side of things.
If I create a webform I can create my own forecasts, but I'd like to be able to visualize that and incorporate variables so the user can see impact in real-time0 -
1. Model Setup with ARIMA (Exogenous Variables) or Random Forest
- ARIMA with Exogenous Variables (ARIMAX): ARIMA can accept external (exogenous) variables in addition to time series data. You can set up an ARIMAX model using Python in Domo's data science tool (like Jupyter Workspaces or Python tile).
- In Python, when fitting an ARIMAX model, use the
statsmodels
library'sSARIMAX
method, and pass your exogenous variables. - After training the model, you can store the forecast results in a dataset in Domo.
- In Python, when fitting an ARIMAX model, use the
- Random Forest for Forecasting: You can also use Random Forest Regressors (or other models like XGBoost) for forecasting if you have explanatory variables. After training the model in a Python tile, you can output the predictions to a dataset.
2. User Input for Exogenous Variables
- Webform for Input: You can create a Domo Webform for user input. This webform could capture variables like economic indicators, marketing spend, or any other factors that affect your forecast.
- These inputs will be stored in a dataset that can be dynamically updated as users enter new values.
- Domo Variables: Domo introduced a Variables feature that allows users to create variables on a dashboard and use them to dynamically adjust visualizations. You can create variables that correspond to the exogenous/explanatory variables used in your ARIMAX or Random Forest model. Users can interact with these variables using dropdowns, sliders, or text inputs on the dashboard.
3. Real-Time Forecast with Python Integration
- After obtaining user input (either through a webform or Domo Variables), you need to link these inputs back into your forecast model.
- In Python, you can fetch this data dynamically (e.g., pulling the most recent inputs from the webform dataset or variable inputs).
- Update your model or create a new prediction based on these inputs, then write the forecast results to a new dataset.
- This dataset can then be visualized on the dashboard, showing the forecast based on the user's custom inputs in real-time.
** Was this post helpful? Click Agree or Like below. **
** Did this solve your problem? Accept it as a solution! **0 - ARIMA with Exogenous Variables (ARIMAX): ARIMA can accept external (exogenous) variables in addition to time series data. You can set up an ARIMAX model using Python in Domo's data science tool (like Jupyter Workspaces or Python tile).
Categories
- All Categories
- 2K Product Ideas
- 2K Ideas Exchange
- 1.6K Connect
- 1.3K Connectors
- 311 Workbench
- 6 Cloud Amplifier
- 9 Federated
- 3.8K Transform
- 656 Datasets
- 115 SQL DataFlows
- 2.2K Magic ETL
- 811 Beast Mode
- 3.3K Visualize
- 2.5K Charting
- 80 App Studio
- 45 Variables
- 771 Automate
- 190 Apps
- 481 APIs & Domo Developer
- 77 Workflows
- 23 Code Engine
- 36 AI and Machine Learning
- 19 AI Chat
- AI Playground
- AI Projects and Models
- 17 Jupyter Workspaces
- 410 Distribute
- 120 Domo Everywhere
- 280 Scheduled Reports
- 10 Software Integrations
- 142 Manage
- 138 Governance & Security
- 8 Domo Community Gallery
- 48 Product Releases
- 12 Domo University
- 5.4K Community Forums
- 41 Getting Started
- 31 Community Member Introductions
- 114 Community Announcements
- 4.8K Archive