It is possible to call the Domo powerpoint plugin with the API ?
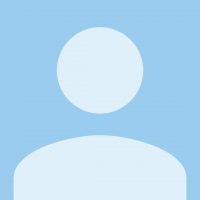
Hi !
I’m looking to run the DOMO powerpoint plugin using a script. Is there a way to do this? An API call to the DOMo plugin?
Basically, the script will need to update the powerpoint based on user-defined filters in a third-party application.
Is that even possible?
Best Answer
-
The PowerPoint plugin is designed to work interactively with PowerPoint so I'm not sure you could do it that way. But you can probably use Python to extract the data from Domo via the Domo API. Then process it according to whatever your needs are such as filtering, aggregating, etc. Then update the PowerPoint with Python.
import requests
#Define your Domo API credentials and dataset ID client_id = 'YOUR_CLIENT_ID'
client_secret = 'YOUR_CLIENT_SECRET'
dataset_id = 'YOUR_DATASET_ID'
#Get access token auth_response = requests.post(
'https://api.domo.com/oauth/token',
data={
'grant_type': 'client_credentials',
'scope': 'data'
},
auth=(client_id, client_secret)
)
auth_data = auth_response.json()
access_token = auth_data['access_token']
#Get data from Domo data_response = requests.get(
f'https://api.domo.com/v1/datasets/{dataset_id}/data',
headers={
'Authorization': f'Bearer {access_token}'
}
)
data = data_response.json()Update PowerPoint would be something like this
from pptx import Presentation
#Create a presentation object prs = Presentation()
#Add a slide with a title and subtitle slide_layout = prs.slide_layouts[0]
slide = prs.slides.add_slide(slide_layout)
title = slide.shapes.title
subtitle = slide.placeholders[1]
title.text = "Title Slide"
subtitle.text = "Subtitle here"
#Add another slide with a title and content slide_layout = prs.slide_layouts[1]
slide = prs.slides.add_slide(slide_layout)
title = slide.shapes.title
title.text = "Content Slide"
#Add data to the slide (e.g., a table) rows = len(data)
cols = len(data[0])
table = slide.shapes.add_table(rows, cols, 0, 0, prs.slide_width, prs.slide_height).table
#Set column names for col_idx, col_name in enumerate(data[0]):
table.cell(0, col_idx).text = col_name
#Fill the table with data for row_idx, row_data in enumerate(data[1:], start=1):
for col_idx, cell_data in enumerate(row_data):
table.cell(row_idx, col_idx).text = str(cell_data)
#Save the presentation prs.save('output_presentation.pptx')** Was this post helpful? Click Agree or Like below. **
** Did this solve your problem? Accept it as a solution! **0
Answers
-
The PowerPoint plugin is designed to work interactively with PowerPoint so I'm not sure you could do it that way. But you can probably use Python to extract the data from Domo via the Domo API. Then process it according to whatever your needs are such as filtering, aggregating, etc. Then update the PowerPoint with Python.
import requests
#Define your Domo API credentials and dataset ID client_id = 'YOUR_CLIENT_ID'
client_secret = 'YOUR_CLIENT_SECRET'
dataset_id = 'YOUR_DATASET_ID'
#Get access token auth_response = requests.post(
'https://api.domo.com/oauth/token',
data={
'grant_type': 'client_credentials',
'scope': 'data'
},
auth=(client_id, client_secret)
)
auth_data = auth_response.json()
access_token = auth_data['access_token']
#Get data from Domo data_response = requests.get(
f'https://api.domo.com/v1/datasets/{dataset_id}/data',
headers={
'Authorization': f'Bearer {access_token}'
}
)
data = data_response.json()Update PowerPoint would be something like this
from pptx import Presentation
#Create a presentation object prs = Presentation()
#Add a slide with a title and subtitle slide_layout = prs.slide_layouts[0]
slide = prs.slides.add_slide(slide_layout)
title = slide.shapes.title
subtitle = slide.placeholders[1]
title.text = "Title Slide"
subtitle.text = "Subtitle here"
#Add another slide with a title and content slide_layout = prs.slide_layouts[1]
slide = prs.slides.add_slide(slide_layout)
title = slide.shapes.title
title.text = "Content Slide"
#Add data to the slide (e.g., a table) rows = len(data)
cols = len(data[0])
table = slide.shapes.add_table(rows, cols, 0, 0, prs.slide_width, prs.slide_height).table
#Set column names for col_idx, col_name in enumerate(data[0]):
table.cell(0, col_idx).text = col_name
#Fill the table with data for row_idx, row_data in enumerate(data[1:], start=1):
for col_idx, cell_data in enumerate(row_data):
table.cell(row_idx, col_idx).text = str(cell_data)
#Save the presentation prs.save('output_presentation.pptx')** Was this post helpful? Click Agree or Like below. **
** Did this solve your problem? Accept it as a solution! **0 -
Agree with @ArborRose on this one. In fact, I had essentially the same response written out, but I guess I forgot to hit "Post". Anyways, just stopping by to say that the path Arbor suggested is the same route I would likely take if I was trying to do this.
David Cunningham
** Was this post helpful? Click Agree 😀, Like 👍️, or Awesome ❤️ below **
** Did this solve your problem? Accept it as a solution! ✔️**0
Categories
- All Categories
- 2K Product Ideas
- 2K Ideas Exchange
- 1.6K Connect
- 1.3K Connectors
- 308 Workbench
- 6 Cloud Amplifier
- 10 Federated
- 3.8K Transform
- 660 Datasets
- 117 SQL DataFlows
- 2.2K Magic ETL
- 815 Beast Mode
- 3.3K Visualize
- 2.5K Charting
- 84 App Studio
- 46 Variables
- 780 Automate
- 191 Apps
- 482 APIs & Domo Developer
- 84 Workflows
- 23 Code Engine
- 41 AI and Machine Learning
- 21 AI Chat
- 2 AI Projects and Models
- 18 Jupyter Workspaces
- 414 Distribute
- 122 Domo Everywhere
- 281 Scheduled Reports
- 11 Software Integrations
- 146 Manage
- 142 Governance & Security
- 8 Domo Community Gallery
- 49 Product Releases
- 12 Domo University
- 5.4K Community Forums
- 41 Getting Started
- 31 Community Member Introductions
- 115 Community Announcements
- 4.8K Archive