"We're sorry but the system can't do that right now." - Connector Builder
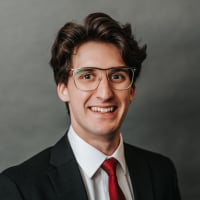
I'm trying to build a custom connector to draw data from the College Scorecard website. I'm using Javascript to write the data processing script and haven't been receiving any errors with my code. However, when I run the script, a new page opens that says "We're sorry but the system can't do that right now." When the script runs, I'll see the "Sending Data, Creating Dataset, and Success!" updates next to the console, but the page opens regardless and I don't have access to the data. Any help or advice would be appreciated.
Best Answer
-
Verify permissions in the API.
Check end pointsconst fetch = require('node-fetch'); const apiKey = 'YOUR_API_KEY';
const apiUrl = https://api.data.gov/ed/collegescorecard/v1/schools?api_key=${apiKey}; async function fetchData() {
try {
const response = await fetch(apiUrl);
if (!response.ok) {
throw new Error(HTTP error! Status: ${response.status});
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
} fetchData();Check for rate limits in the API.
const fetch = require('node-fetch'); const apiKey = 'YOUR_API_KEY';
const apiUrl = https://api.data.gov/ed/collegescorecard/v1/schools?api_key=${apiKey}; async function fetchData() {
try {
const response = await fetch(apiUrl);
if (!response.ok) {
if (response.status === 429) {
console.error('Rate limit exceeded. Try again later.');
} else {
console.error(HTTP error! Status: ${response.status});
}
return;
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
} fetchData();** Was this post helpful? Click Agree or Like below. **
** Did this solve your problem? Accept it as a solution! **1
Answers
-
Verify permissions in the API.
Check end pointsconst fetch = require('node-fetch'); const apiKey = 'YOUR_API_KEY';
const apiUrl = https://api.data.gov/ed/collegescorecard/v1/schools?api_key=${apiKey}; async function fetchData() {
try {
const response = await fetch(apiUrl);
if (!response.ok) {
throw new Error(HTTP error! Status: ${response.status});
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
} fetchData();Check for rate limits in the API.
const fetch = require('node-fetch'); const apiKey = 'YOUR_API_KEY';
const apiUrl = https://api.data.gov/ed/collegescorecard/v1/schools?api_key=${apiKey}; async function fetchData() {
try {
const response = await fetch(apiUrl);
if (!response.ok) {
if (response.status === 429) {
console.error('Rate limit exceeded. Try again later.');
} else {
console.error(HTTP error! Status: ${response.status});
}
return;
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
} fetchData();** Was this post helpful? Click Agree or Like below. **
** Did this solve your problem? Accept it as a solution! **1 -
ArborRose - Thanks! This was the issue.
0
Categories
- All Categories
- 1.9K Product Ideas
- 1.9K Ideas Exchange
- 1.6K Connect
- 1.3K Connectors
- 302 Workbench
- 6 Cloud Amplifier
- 9 Federated
- 2.9K Transform
- 104 SQL DataFlows
- 636 Datasets
- 2.2K Magic ETL
- 3.9K Visualize
- 2.5K Charting
- 761 Beast Mode
- 65 App Studio
- 42 Variables
- 701 Automate
- 182 Apps
- 457 APIs & Domo Developer
- 52 Workflows
- 10 DomoAI
- 39 Predict
- 16 Jupyter Workspaces
- 23 R & Python Tiles
- 401 Distribute
- 116 Domo Everywhere
- 277 Scheduled Reports
- 8 Software Integrations
- 132 Manage
- 129 Governance & Security
- 8 Domo Community Gallery
- 38 Product Releases
- 12 Domo University
- 5.4K Community Forums
- 40 Getting Started
- 30 Community Member Introductions
- 111 Community Announcements
- 4.8K Archive