Difference of values between 2 months
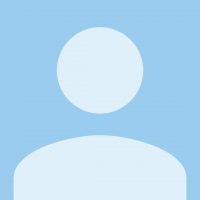
I have a value column named 'Value' and a month column named 'Month'. I want to create a beast mode to calculate the difference between values of the last two months.
So if we have two months in the dataset - Nov 23 and Oct 23. I will want to calculate the difference in value between both months. It is a month-to-month difference. If there is no new month in the dataset like if we are in the Jan 24 month but in the dataset there is no data for Jan 24 then it should print 0.
Best Answers
-
If you have one value per month you can utilize a LAG window function. I've written up about these here:
Alternatively you can restructure your data so that you have a period type (Current, Last Month) and a specific value for your months then utilize some beast modes to calculate the difference. Here's another write up on this method:
A third option is to utilize an ETL and join the data to itself based on a month prior using a formula tile:
`Month` - INTERVAL 1 MONTH
Then join it back onto itself based on the one month prior and the current month and rename your one month prior values as new columns
**Was this post helpful? Click Agree or Like below**
**Did this solve your problem? Accept it as a solution!**0 -
Let's assume that the "Month" column has the first date of the month referenced.
The Beast Mode you will want to use is the following:
sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) - sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)
if you want to return 0 instead of null for empty values, simply wrap your function in a case expression:
example: case when (function) is null then 0 else (function) end
case when (sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) - sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)) is null then 0 else (sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) - sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)) end
If you want to return current month - prior month instead of prior month - current month, just flip the order of the subtraction in the function:
(-1 * sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) )+ sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)
Full version using flipped subtraction order:
case when ((-1 * sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) )+ sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)) is null then 0 else (f(-1 * sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) )+ sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)) end
I have never tried to use interval 0 month with date_sub, so there's a chance that it might not work. Nothing ventured, nothing gained!
0
Answers
-
If you have one value per month you can utilize a LAG window function. I've written up about these here:
Alternatively you can restructure your data so that you have a period type (Current, Last Month) and a specific value for your months then utilize some beast modes to calculate the difference. Here's another write up on this method:
A third option is to utilize an ETL and join the data to itself based on a month prior using a formula tile:
`Month` - INTERVAL 1 MONTH
Then join it back onto itself based on the one month prior and the current month and rename your one month prior values as new columns
**Was this post helpful? Click Agree or Like below**
**Did this solve your problem? Accept it as a solution!**0 -
Let's assume that the "Month" column has the first date of the month referenced.
The Beast Mode you will want to use is the following:
sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) - sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)
if you want to return 0 instead of null for empty values, simply wrap your function in a case expression:
example: case when (function) is null then 0 else (function) end
case when (sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) - sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)) is null then 0 else (sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) - sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)) end
If you want to return current month - prior month instead of prior month - current month, just flip the order of the subtraction in the function:
(-1 * sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) )+ sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)
Full version using flipped subtraction order:
case when ((-1 * sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) )+ sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)) is null then 0 else (f(-1 * sum(case when `Month` = date_sub(`Month`, interval 1 month) then `Value` end) )+ sum(case when `Month` = date_sub(`Month`, interval 0 month) then `Value` end)) end
I have never tried to use interval 0 month with date_sub, so there's a chance that it might not work. Nothing ventured, nothing gained!
0
Categories
- All Categories
- 2K Product Ideas
- 2K Ideas Exchange
- 1.6K Connect
- 1.3K Connectors
- 310 Workbench
- 7 Cloud Amplifier
- 9 Federated
- 3K Transform
- 114 SQL DataFlows
- 654 Datasets
- 2.2K Magic ETL
- 4K Visualize
- 2.5K Charting
- 798 Beast Mode
- 78 App Studio
- 44 Variables
- 757 Automate
- 188 Apps
- 480 APIs & Domo Developer
- 72 Workflows
- 17 DomoAI
- 40 Predict
- 17 Jupyter Workspaces
- 23 R & Python Tiles
- 408 Distribute
- 119 Domo Everywhere
- 279 Scheduled Reports
- 10 Software Integrations
- 141 Manage
- 137 Governance & Security
- 8 Domo Community Gallery
- 47 Product Releases
- 12 Domo University
- 5.4K Community Forums
- 41 Getting Started
- 31 Community Member Introductions
- 114 Community Announcements
- 4.8K Archive