Not sure what this AWS Lambda error I'm getting from trying to extract domo datasets
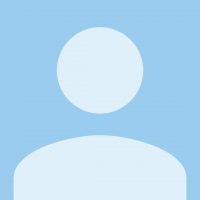
What does this error from AWS Lambda mean?
[
"{\"status\":404,\"statusReason\":\"Not Found\",\"toe\":\"FJACDOT2HE-250JI-8N0AH\"}"
]
Best Answer
-
My message seems to have disappeared.... At risk of this showing up twice I'm posting it again.
-----------------------
I took your code and tested it out using the Base64 Authorization as documented in the developer documentation instead of how you did it and it worked. (Note, I ran this locally, not in AWS but that shouldn't make a difference since you're just returning the HTTP response)
import requests
def get_dataset(id):
# Get Access Token and Domo API Response
base64auth = 'InsertBase64_ID+Secret'
r = requests.get("https://api.domo.com/oauth/token?grant_type=client_credentials&scope=data",
headers={'Authorization': 'Basic ' + base64auth})
tkn_response = r.json()
tkn = tkn_response['access_token']
headers = {
'Content-Type': 'application/json',
'Authorization': 'bearer ' + tkn,
'content-encoding': 'utf-8'
}
url = "https://api.domo.com/v1/datasets/" + str(id) + "/data"
resp = requests.get(url, headers=headers)
# convert csv string to list
print(resp.text.splitlines())
return resp.text.splitlines()
get_dataset('78c41cbc-43d4-4dba-a638-86b67cd86356')
Output (printed)['667b327f-67f6-4d74-9d4a-5d0697b938cf, General Ledger Detail Lookup,Travis Simpson,2019-01-11 14:21:01,2016-12-07 17:53:47,2019-0............]
You can use this to help you with that type of authorization https://developer.domo.com/explorer#!/auth
**Make sure to like any users posts that helped you and accept the ones who solved your issue.**1
Answers
-
Can you provide the Lambda code? Is this a response from the Domo API that you're getting with a call from a Lambda function?
**Make sure to like any users posts that helped you and accept the ones who solved your issue.**0 -
Here's the code I'm using. The references is just AWS and DOMO API stuff.
import requests
import reference
def get_dataset(self, id):
# Get Access Token and Domo API Response
r = requests.get("https://api.domo.com/oauth/token?grant_type=client_credentials&scope=data",
auth=(reference.CLIENT_ID, reference.CLIENT_SECRET))
tkn_response = r.json()
tkn = tkn_response['access_token']
headers = {
'Content-Type': 'application/json',
'Authorization': 'bearer ' + tkn,
'content-encoding': 'utf-8'
}
url = "https://api.domo.com/v1/datasets/" + str(id) + "/data"
resp = requests.get(url, headers=headers)
# convert csv string to list
return resp.text.splitlines()1 -
I took your code and tested it out using the Base64 Authorization as documented in the developer documentation instead of how you did it and it worked. (Note, I ran this locally, not in AWS but that shouldn't make a difference since you're just returning the HTTP response)
import requests
def get_dataset(id):
# Get Access Token and Domo API Response
base64auth = 'InsertBase64_ID+Secret'
r = requests.get("https://api.domo.com/oauth/token?grant_type=client_credentials&scope=data",
headers={'Authorization': 'Basic ' + base64auth})
tkn_response = r.json()
tkn = tkn_response['access_token']
headers = {
'Content-Type': 'application/json',
'Authorization': 'bearer ' + tkn,
'content-encoding': 'utf-8'
}
url = "https://api.domo.com/v1/datasets/" + str(id) + "/data"
resp = requests.get(url, headers=headers)
# convert csv string to list
print(resp.text.splitlines())
return resp.text.splitlines()
get_dataset('78c41cbc-43d4-4dba-a638-86b67cd86356')Output (printed)
['667b327f-67f6-4d74-9d4a-5d0697b938cf, General Ledger Detail Lookup,Travis Simpson,2019-01-11 14:21:01,2016-12-07 17:53:47,2019-0............]
You can use this to help you with that type of authorization https://developer.domo.com/explorer#!/auth
**Make sure to like any users posts that helped you and accept the ones who solved your issue.**0 -
My message seems to have disappeared.... At risk of this showing up twice I'm posting it again.
-----------------------
I took your code and tested it out using the Base64 Authorization as documented in the developer documentation instead of how you did it and it worked. (Note, I ran this locally, not in AWS but that shouldn't make a difference since you're just returning the HTTP response)
import requests
def get_dataset(id):
# Get Access Token and Domo API Response
base64auth = 'InsertBase64_ID+Secret'
r = requests.get("https://api.domo.com/oauth/token?grant_type=client_credentials&scope=data",
headers={'Authorization': 'Basic ' + base64auth})
tkn_response = r.json()
tkn = tkn_response['access_token']
headers = {
'Content-Type': 'application/json',
'Authorization': 'bearer ' + tkn,
'content-encoding': 'utf-8'
}
url = "https://api.domo.com/v1/datasets/" + str(id) + "/data"
resp = requests.get(url, headers=headers)
# convert csv string to list
print(resp.text.splitlines())
return resp.text.splitlines()
get_dataset('78c41cbc-43d4-4dba-a638-86b67cd86356')
Output (printed)['667b327f-67f6-4d74-9d4a-5d0697b938cf, General Ledger Detail Lookup,Travis Simpson,2019-01-11 14:21:01,2016-12-07 17:53:47,2019-0............]
You can use this to help you with that type of authorization https://developer.domo.com/explorer#!/auth
**Make sure to like any users posts that helped you and accept the ones who solved your issue.**1 -
So really dumb question but the line where you have:
base64auth = 'InsertBase64_ID+Secret'
is that where I sub out the InsertBase64_ID with the Client ID and the Secret with Client Secret?
1 -
You would want to get the base64 encoded version of your client secret and client ID combined. You could do this in code but I found it easiest to go to that Domo developer site I mentioned in the comment and generate it there. If you scroll down on that page it has a spot you can fill in your Client ID and Secret and on the right it should show you the Base64 version of those two things combined. You could do something like import base64 and run a function from that to do it yourself but I say just go to https://developer.domo.com/explorer#!/auth and do it there, screenshot below with an altered CLIENT ID + SECRET
**Make sure to like any users posts that helped you and accept the ones who solved your issue.**0 -
Ah ok thank you so much! I encountered an addditional problem after this though. I got this error:
{
"errorMessage": "get_dataset() takes 1 positional argument but 2 were given",
"errorType": "TypeError",
"stackTrace": [
" File \"/var/runtime/bootstrap.py\", line 119, in handle_event_request\n response = request_handler(event, lambda_context)\n"
]
}People on stackoverflow were saying that I need to add self as an additonal parameter for the function. But then it throws this error and now I'm stuck at how to get past it:
{
"errorMessage": "get_dataset() missing 1 required positional argument: 'id'",
"errorType": "TypeError",
"stackTrace": [
" File \"/var/lang/lib/python3.7/imp.py\", line 234, in load_module\n return load_source(name, filename, file)\n",
" File \"/var/lang/lib/python3.7/imp.py\", line 171, in load_source\n module = _load(spec)\n",
" File \"<frozen importlib._bootstrap>\", line 696, in _load\n",
" File \"<frozen importlib._bootstrap>\", line 677, in _load_unlocked\n",
" File \"<frozen importlib._bootstrap_external>\", line 728, in exec_module\n",
" File \"<frozen importlib._bootstrap>\", line 219, in _call_with_frames_removed\n",
" File \"/var/task/get_domo_dataset.py\", line 25, in <module>\n get_dataset('78c41cbc-43d4-4dba-a638-86b67cd86356')\n"
]
}0 -
Ah the classic solve one thing just to find out something else is also wrong.
I did change in mine to remove self only because to test it for you I didn't need that. Did you try it with and without it in you're real code? Also what exactly is the end product of what you're producing? I'm interested. My guess seeing as how it's bootstrap is you're presenting the data on the web? So like someone goes to a page and or does an action and it makes a call via API Gateway + Lambda and returns the data which is then represented on the page? Generally Self would be inherited from a parent class or something however if you are just making it a single function without a parent class you may or may not need it (I'm an amateur coder myself so don't take my word as authority). It does sound like the Self is messing it up because it's expecting you to supply the dataset ID and a value for Self
**Make sure to like any users posts that helped you and accept the ones who solved your issue.**0
Categories
- All Categories
- 1.9K Product Ideas
- 1.9K Ideas Exchange
- 1.6K Connect
- 1.3K Connectors
- 306 Workbench
- 6 Cloud Amplifier
- 9 Federated
- 3K Transform
- 112 SQL DataFlows
- 649 Datasets
- 2.2K Magic ETL
- 4K Visualize
- 2.5K Charting
- 788 Beast Mode
- 78 App Studio
- 43 Variables
- 744 Automate
- 187 Apps
- 474 APIs & Domo Developer
- 67 Workflows
- 16 DomoAI
- 40 Predict
- 17 Jupyter Workspaces
- 23 R & Python Tiles
- 406 Distribute
- 117 Domo Everywhere
- 279 Scheduled Reports
- 10 Software Integrations
- 139 Manage
- 136 Governance & Security
- 8 Domo Community Gallery
- 44 Product Releases
- 12 Domo University
- 5.4K Community Forums
- 41 Getting Started
- 31 Community Member Introductions
- 113 Community Announcements
- 4.8K Archive