Creating an HMAC and pushing data to a JSON Webhook connector with a secret applied
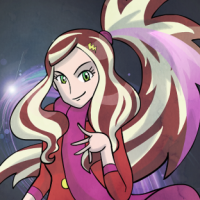
It can be a little confusing when trying to setup the JSON Webhook connector with a secret applied and push data successfully.
The biggest hurdle can often be creating the HMAC to pass in through the header. Depending on the source that you're pushing from or what programming language you're using will change the setup process and/or the libraries used. But the format of the HMAC is the same. The secret will be used as the key in the HMAC, your JSON body (data) will be the included message, and then either SHA1, SHA256 or SHA512 for the hash algorithm.
I've created an example written in Python of the process of creating an HMAC and pushing the data into Domo through the JSON Webhook connector with the secret "webhookSecret" applied to the connector.
import requests import hashlib import hmac def jsonWebhookSecretExample(): url = 'https://example.domo.com/api/iot/v1/webhook/data/aseKEdjweksKSSPwasjdflKEecklsClsjeQ' jsonStr = '{"data": [{"name": "Ashley", "age": 22}, {"name": "Ben", "age": 24}, {"name": "Melissa", "age": 31}, {"name": "Eddy", "age": 29}]}' key = 'webhookSecret' byteKey = bytes(key, 'UTF-8') message = jsonStr.encode() hashed = hmac.new(byteKey, message, hashlib.sha1) response = requests.post(url, headers={'X-Hub-Signature': hashed.hexdigest(), 'Content-Type': 'application/json'})
Comments
-
Thanks so much for this post. Been trying to work this out. I notice there is not "data="/payload in the post request. Is this right? I am getting a http 200 back but data doesn't seem to be adding to the dataset.
0 -
For me to get this to work I had to add the "data=" parameter in the request:
response = requests.post(url, headers={'X-Hub-Signature': hashed.hexdigest(), 'Content-Type': 'application/json'}, data=jsonStr)
0 -
Setting up the JSON Webhook connector with a secret can be tricky, especially when generating the HMAC correctly. If you're working on IoT integrations, this guide might be useful: https://blog.urlaunched.com/wearable-iot-development-for-healthcare-startups/
0
Categories
- All Categories
- 2K Product Ideas
- 2K Ideas Exchange
- 1.6K Connect
- 1.3K Connectors
- 308 Workbench
- 6 Cloud Amplifier
- 10 Federated
- 3.8K Transform
- 660 Datasets
- 117 SQL DataFlows
- 2.2K Magic ETL
- 815 Beast Mode
- 3.3K Visualize
- 2.5K Charting
- 84 App Studio
- 46 Variables
- 780 Automate
- 191 Apps
- 482 APIs & Domo Developer
- 84 Workflows
- 23 Code Engine
- 41 AI and Machine Learning
- 21 AI Chat
- 2 AI Projects and Models
- 18 Jupyter Workspaces
- 414 Distribute
- 122 Domo Everywhere
- 281 Scheduled Reports
- 11 Software Integrations
- 146 Manage
- 142 Governance & Security
- 8 Domo Community Gallery
- 49 Product Releases
- 12 Domo University
- 5.4K Community Forums
- 41 Getting Started
- 31 Community Member Introductions
- 115 Community Announcements
- 4.8K Archive